Overview
The purpose of this project portfolio is to document my contributions I have made for the project, SuperTA.
SuperTA is a desktop app for Teaching Assistants and Professors of NUS School of Computing to manage their tutorials and students. This app has been developed from an existing software product by my CS2103T team and helps TAs with their administrative tasks. It is optimized for those who prefer to work with a Command Line Interface (CLI) while still having the benefits of a Graphical User Interface (GUI). It is written in Java, and it is equipped with various Continuous Integration/ Deployment tools such as AppVeyor and Travis CI.
Some significant features of SuperTA include:
-
Creating of tutorial groups
-
Adding of students into tutorial groups
-
Marking attendance of students
-
Grading assignments of students
-
Giving feedback to students
Summary of contributions
-
Major enhancement: added the ability to create attendance sessions and mark attendance for students (Pull requests #97, #131)
-
What it does: This allows the teaching assistant to create attendance sessions for each tutorial class, and subsequently mark the attendance of students in those sessions.
-
Justification: This feature is an essential part of SuperTA, since taking attendance of students is definitely a feature required by teaching assistants in classes.
-
Highlights: This enhancement is able to take in multiple student IDs as user input, allowing the attendance of multiple students to be marked a the same time. Also, this enhancement involves creating the Presence enumeration to properly encapsulate the attendance of students using fixed and constant values. The implementation of these commands needed to account for various exceptions, such as when the tutorial group is invalid, when the session does not exist, as well as when the attendance of students are already marked.
-
-
Major enhancement: added the ability to write feedback to a student (Pull request #43)
-
What it does: allows the teaching assistant to create feedback for a student for viewing in the future.
-
Justification: This feature improves the usability of the SuperTA client as TAs are better able to craft personalized feedback for students to help with their studies.
-
Highlights: This enhancement involves modifying the Student class and adding a new data field, Feedback to it. New feedback can also be easily added to a Student without overwriting existing feedback, as feedback objects have been modelled as array elements within the student object instead.
-
-
Minor enhancement: Modified the view command that allows the TA to view past feedback given to a student. (Pull request #83)
-
Minor enhancement: Modified the parser to be able to accept both lowercase and uppercase Student IDs. (Pull request #133)
-
Code contributed: (RepoSense collated code)
-
Other contributions:
-
Project management:
-
Reposense: Created config.json file and modified author names to ensure code is correctly attributed
-
-
Enhancements to existing features:
-
Documentation:
-
Slight modifications to feedback command in the User Guide: #83
-
-
Community:
-
Contributions to the User Guide
Given below are sections I contributed to the User Guide. They showcase my ability to write documentation targeting end-users. |
Feedback to Student: feedback
Adds feedback to a student with a specified Student ID.
Format: feedback id/STUDENT-ID f/FEEDBACK
Examples:
-
feedback id/A1234566T f/Is generally attentive during class. However, needs to speak up more.
Adds the given feedbackIs generally attentive during class. However, needs to speak up more.
to the student with Student IDA1234566T
.
Create Attendance: create-attendance
Creates an attendance listing for a specific tutorial group, identified by its ID.
Format: create-attendance tg/TUTORIAL-GROUP-ID n/SESSION-NAME
Examples:
-
create-attendance tg/1 n/W4 Tutorial
Creates an attendance namedW4 Tutorial
for the tutorial group with an ID of1
.
Mark Attendance: mark-attendance
Marks attendance for students from a specific tutorial group and session name, identified by its ID and name.
Format: mark-attendance tg/TUTORIAL-GROUP-ID n/SESSION-NAME st/STUDENT-ID …
Examples:
-
mark-attendance tg/1 n/W4 Tutorial st/A1234567T st/A0123456Y
Marks students with IDsA1234567T
andA0123456Y
as present in tutorial session namedW4 Tutorial
for the tutorial group with an ID of1
.
Contributions to the Developer Guide
Given below are sections I contributed to the Developer Guide. They showcase my ability to write technical documentation and the technical depth of my contributions to the project. |
Mark Attendance feature
Current Implementation
The mark-attendance
command is facilitated by the MarkAttendanceCommand
class.
It extends the abstract class Command
and is supported by MarkAttendanceCommandParser
,
and changes made to the Model
are done through Model#markAttendance()
.
The mark-attendance
command is executed in the following way:
-
LogicManager
callsparseCommand()
ofSuperTaClientParser
, with the user input as the argument. -
SuperTaClientParser
invokesparse()
ofMarkAttendanceCommandParser
. -
If arguments are valid,
parse
extractstutorialGroupId
string,sessionName
and set ofstudentId
strings for parsing into aSession
andSet<StudentId>
to instantiate aMarkAttendanceCommand
. -
The
MarkAttendanceCommand
is returned toLogicManager
, which callsexecute()
on it. -
MarkAttendance
callsModel#markAttendance()
usingtgtId
,sessionName
andstudentIdSet
.
The following sequence diagrams of the mark-attendance
command shows the sequence flow from LogicManager
to the ModelManager
:
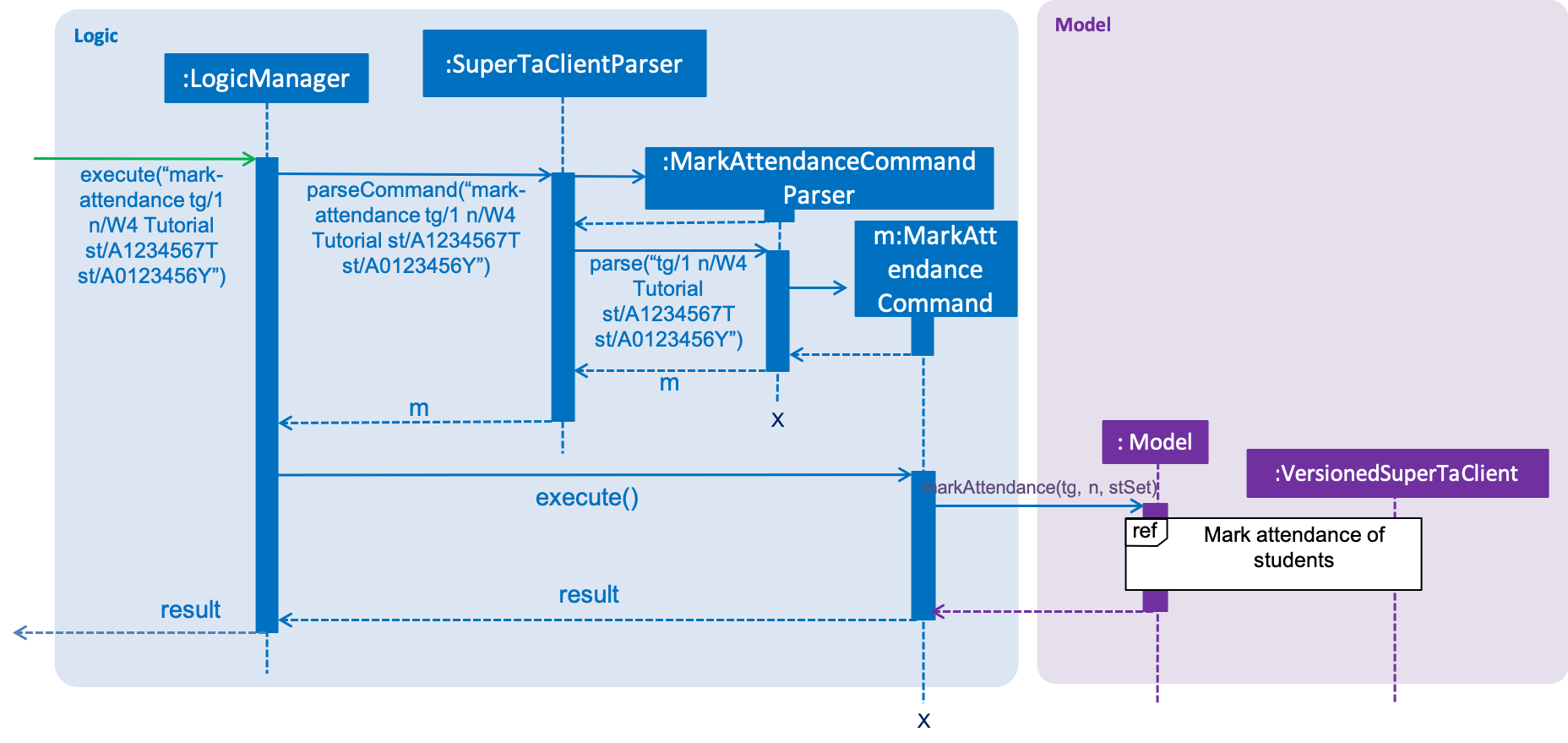
A more in-depth sequence diagram to illustrate how the Model
executes the mark-attendance
command:
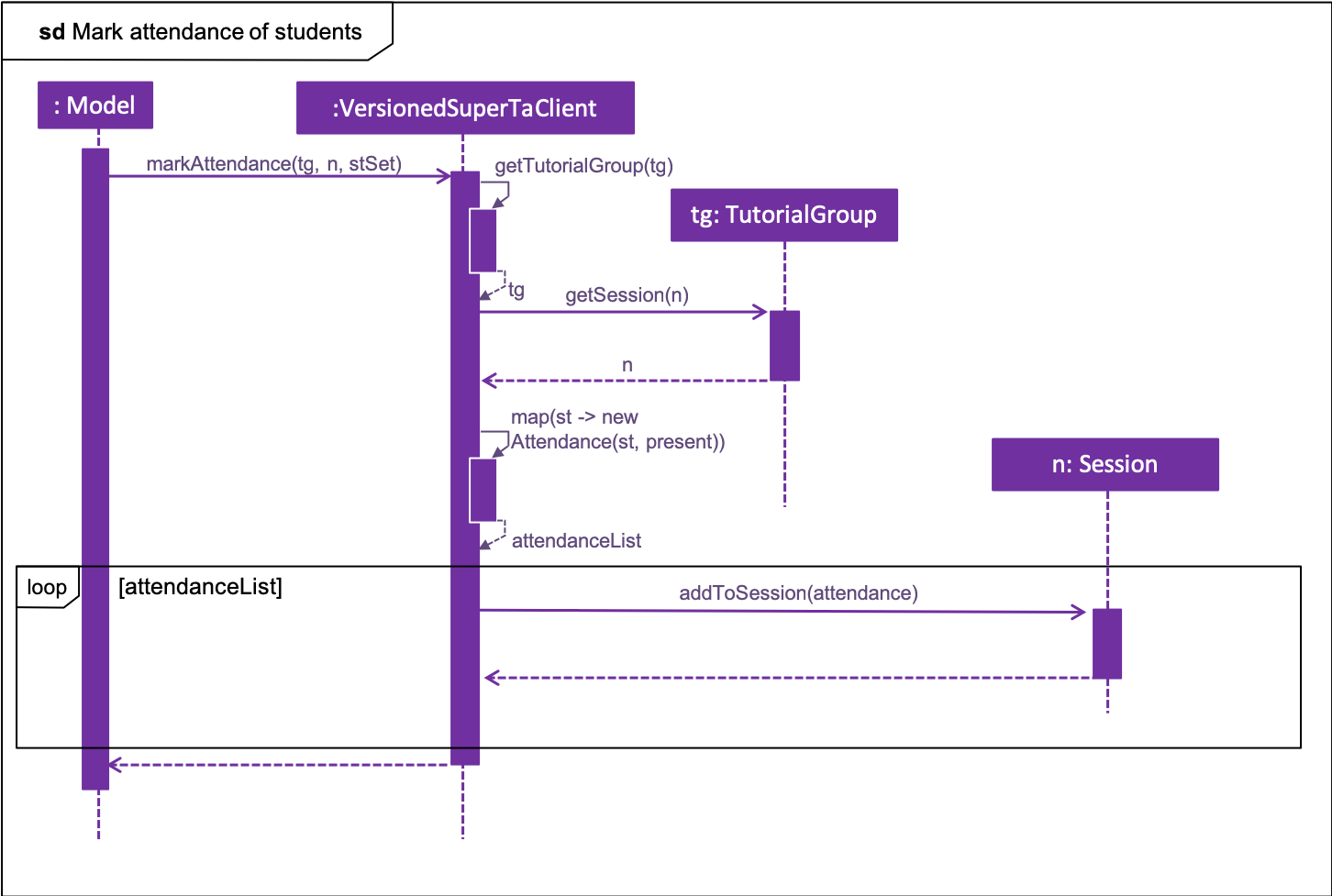
From the in-depth sequence diagram:
-
Model
callsVersionedSuperTaClient#markAttendance
, usingtgtId
,sessionName
andstudentIdSet
. -
VersionedSuperTaClient
self-invokes the methodgetTutorialGroup(tgId)
to obtain the tutorial group specified. -
VersionedSuperTaClient
then callsgetSession(sessionName)
in theTutorialGroup
instance obtained previously. -
VersionedSuperTaClient
then streams the set of student Ids obtained instudentIdSet
, mapping them to newAttendance
objects and collecting them as a list. -
Finally, the
attendanceList
obtained is streamed, with eachAttendance
object being added to theSession
instance obtained in step 3.
Design Considerations
Aspect: Implementation of MarkAttendanceCommand
-
Alternative 1(current choice): Store the tutorial group ID as String field, session name as a
Session
object and student ids as a set ofStudentIds
inMarkAttendanceCommand
.-
Pros: Easy to implement, and parse, with existing parsers built in. Ensures that parsed
Session
andStudentId
objects are valid and abide by constraints. -
Cons: Requires
Session
andStudentId
objects to be instantiated. Also depends onisSameSession()
method andcontainsId()
method inSession
andUniqueStudentList
respectively.
-
-
Alternative 2: Store all parameters of
MarkAttendanceCommand
as String objects, includingStudentIds
as a set of Strings.-
Pros: Easy to implement, will be able to find session and set of student ids by comparing strings.
-
Cons: Cannot ensure parsed session names and student ids are correct, valid and fit constraints.
-
Aspect: Implementation of Attendance
-
Alternative 1(current choice): Store
Attendance
object with an encapsulatedStudentId
object andPresence
enumeration.-
Pros:
StudentId
is assured to be valid, whilePresence
will only be able to take fixed, constant values, -
Cons: Requires a new
Presence
enumeration to be defined, instead of relying on simpler string values.
-
-
Alternative 2: Store presence of student as an integer or string value.
-
Pros: Easy to implement, no need to create additional enumeration.
-
Cons: Cannot ensure that only fixed, valid values will be used when creating
Attendance
objects.
-
Creating an attendance session
-
Creating an attendance session
-
Prerequisites: Have one tutorial group with an ID of
04a
.
View the tutorial group using theview-tutorial-group id/04a
command. -
Test case:
create-attendance tg/04a n/W4 Tutorial
Expected: An attendance session should be created. If you are on the detailed tutorial group screen, the UI should update accordingly. -
Test case:
create-attendance tg/05a n/W4 Tutorial
Expected: An error message should be displayed, saying that there is no such tutorial group. -
Test case:
create-attendance tg/04a n/W4 Tutorial
Expected: An error message should be displayed, saying that the attendance session already exists in the tutorial group.
-
Marking an attendance
-
Marking an attendance
-
Prerequisites: Have one tutorial group with an ID of
04a
, an attendance session with name ofW4 Tutorial
and 3 students with student IDsA0166733Y
,A0123456Y
andA0144582N
in the tutorial group.
View the session in the tutorial group using theview-session tg/04a n/W4 Tutorial
command. -
Test case:
mark-attendance tg/04a n/W4 Tutorial st/A0166733Y
Expected: The student with idA0166733Y
should now be in the 'Attended' column. -
Test case:
mark-attendance tg/04a n/W4 Tutorial st/A0123456Y st/A0144582N
Expected: Both students with idsA0123456Y
andA0144582N
should now be in the 'Attended' column, asmark-attendance
supports marking multiple students. -
Test case: Duplicate ID -
mark-attendance tg/04a n/W4 Tutorial st/A0166733Y
Expected: An error message should be displayed, saying that the student’s attendance is already marked. -
Test case:
mark-attendance tg/05a n/W4 Tutorial st/A0166733Y
Expected: An error message should be displayed, saying that there is no such tutorial group. -
Test case:
mark-attendance tg/04a n/W4 Lab st/A0166733Y
Expected: An error message should be displayed, saying that the session does not exist. -
Test case:
mark-attendance tg/04a n/W4 Tutorial st/A1234560T
Expected: An error message should be displayed, saying that the student is not in the tutorial group.
-