Overview
SuperTA is a class management tool created for School of Computing (SoC) Teaching Assistants (TA) and professors. It enables the TAs and professors to better manage their tutorial classes and streamline trivial process such as attendance marking and assignment marks management.
This project involves a team of Computer Science (CS) undergraduates to develop a software from an existing software product. The 10KoC codebase was originally adapted from Seedu’s AddressBook – Level 4 application. The user of interacts with SuperTA using a CLI, and it has a GUI created with JavaFX.
Summary of contributions
-
Major enhancement: enhancement made to find command (#64)
-
What it does: allows the user to find students in the software and list the students according to the searched keyword.
-
Justification: Previously, user can only search students using name. After the enhancements are made, user can now search students using other parameters such as phone number or student ID. This enhancement improves the product usability as users can now find their desired result more easily.
-
Highlights: Further improvements on the command will be needed in the future as more parameters are added into the software such as tutorial groups and assignment grades.
-
Credits: Most of the codes are adapted from Seedu’s Addressbook application, and further enhancements are made thereafter.
-
-
Minor enhancement: added
UpdateAssignmentCommand
which allow users to edit an existing assignment details (ie assignment title, maximum marks) from an existing tutorial group. (#152) -
Code contributed: (https://nus-cs2103-ay1819s1.github.io/cs2103-dashboard/#=undefined&search=darieca)
-
Other contributions:
Contributions to the User Guide
Given below are sections I contributed to the User Guide. They showcase my ability to write documentation targeting end-users. |
Locating students by keywords: find
Finds and lists all students in the SuperTA client whose information matched with the entered keywords.
Format: find [n/NAME] [p/PHONE] [e/EMAIL] [id/STUDENT-ID]
Examples:
-
find n/John
Returnsjohn
andJohn Doe
-
find p/91234567
Returns student with phone number91234567
-
find e/Johndoe@hotmail.com
Returns student with emailJohndoe@hotmail.com
-
find n/John id/A0123456T
Returnsjohn
andJohn Doe
and student with student idA0123456T
-
find n/Alice n/Hans
ReturnsHans
only
Update an Assignment: update-assignment
Updates an existing assignment’s details such as title and maximum marks.
Format: update-assignment tg/TUTORIAL-GROUP-ID as/OLD-ASSIGNMENT-TITLE
[new_as/NEW-ASSIGNMENT-TITLE] [new_m/NEW-ASSIGNMENT-MAX-MARKS]
Examples:
-
update-assignment tg/04a as/lab1 new_as/lab2
Updates an assignment namedlab1
tolab2
for the tutorial group with an ID of04a
. -
update-assignment tg/04a as/lab1 new_m/50
Updateslab1
assignment maximum marks from40.0
to50.0
marks for the tutorial group with an ID of04a
. -
update-assignment tg/04a as/lab1 new_as/lab2 new_m/50
Updates an assignment namedlab1
and maximum marks of40.0
, to assignment namedlab2
and maximum marks of50.0
for the tutorial group with an ID of04a
.
Contributions to the Developer Guide
Given below are sections I contributed to the Developer Guide. They showcase my ability to write technical documentation and the technical depth of my contributions to the project. |
Find feature
Current Implementation
The find
command is facilitated by the FindCommand
class.
It extends the abstract class Command
,
and it is triggered when the user enters find
into SuperTaClient
.
The sequence diagram below shows the overview when FindCommand
is called:
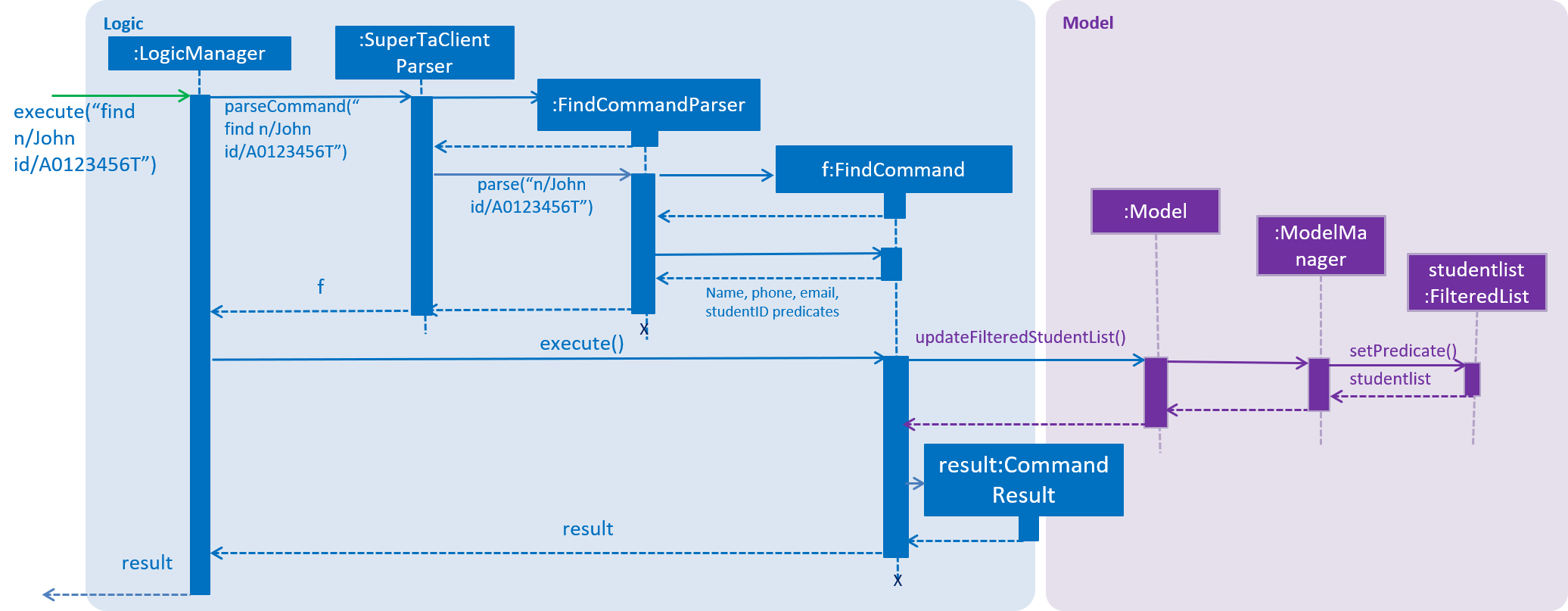
The FindCommand
is implemented as follows:
-
LogicManager
is called to execute the entered argument. -
LogicManager
callsSuperTaClientParser
, and parses the argument through the methodparseCommand()
. -
SuperTaClientParser
calls its respective command parsers of the argument usingparse()
. In this case, theFindCommandParser
is called. -
FindCommandParser
will now parse the arguments and turn intoname
,phone
,email
andstudentID
predicates. These predicates will be passed toFindCommand
. -
When
LogicManager
invokesexecute()
,FindCommand
will callupdateFilteredStudentList()
to the model component and update the student list to the latest results.
Design Considerations
Aspect: How FindCommandParser
parse predicates
-
Alternative 1 (current choice): Each parameter field (ie
name
,phone
) are parsed into its respective predicates (ie.namePredicate
,phonePredicate
).-
Pros: Easy to implement. Predicates are grouped into how they are parsed.
-
Cons: Less user-friendly. Users are required to remember the prefixes for each field.
-
-
Alternative 2: Parse all arguments into a general
string
, then search all the fields and stored into a main predicate.-
Pros: More versatile and user-friendly. The
string
is able to search all the fields. -
Cons: Slightly more tedious as compared to alternative 1. The general
string
needs to parse into respective field objects and predicates for each available parameter.
-
Update Assignment feature
Current Implementation
The update-assignment
command is facilitated by UpdateAssignmentCommand
class.
It extends the abstract class command
,
and it is triggered when the user enters update-assignment
into SuperTaClient
.
Actual changes of this command are made through Model#updateAssignment()
.
The UpdateAssignmentCommand
is implemented as follows:
UpdateAssignmentCommand only works when there is an existing assignment in an existing tutorial group,
and the updated assignment name is not the same as other existing assignment title.
In this implementation, it is assumed that there is a valid tutorial group and a valid existing assignment.
|
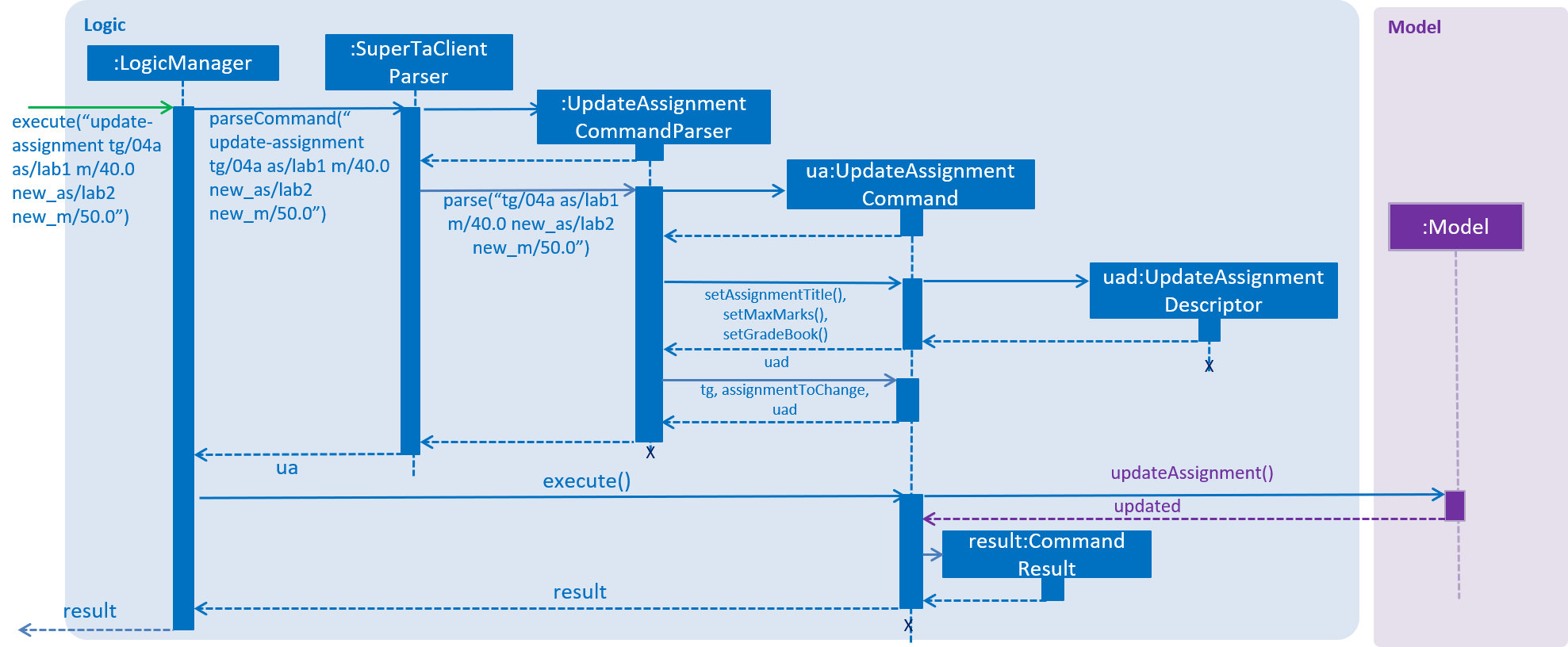
update-assignment
command-
LogicManager
is called to execute the entered argument. -
LogicManager
callsSuperTaClientParser
, and parses the argument through the methodparseCommand()
. -
SuperTaClientParser
calls its respective command parsers of the argument usingparse()
. In this case, theUpdateAssignmentCommandParser()
is called. -
UpdatAassignmentCommandParser
will now parse the arguments. -
UpdateAssignmentCommandParser
will create a newUpdateAssignmentDescriptor
and set the updated assignment details, which later will be passed toUpdateAssignmentCommand
. -
When
LogicManager
invokesexecute()
,UpdateAssignmentCommand
will callupdateAssignment()
to the model component.
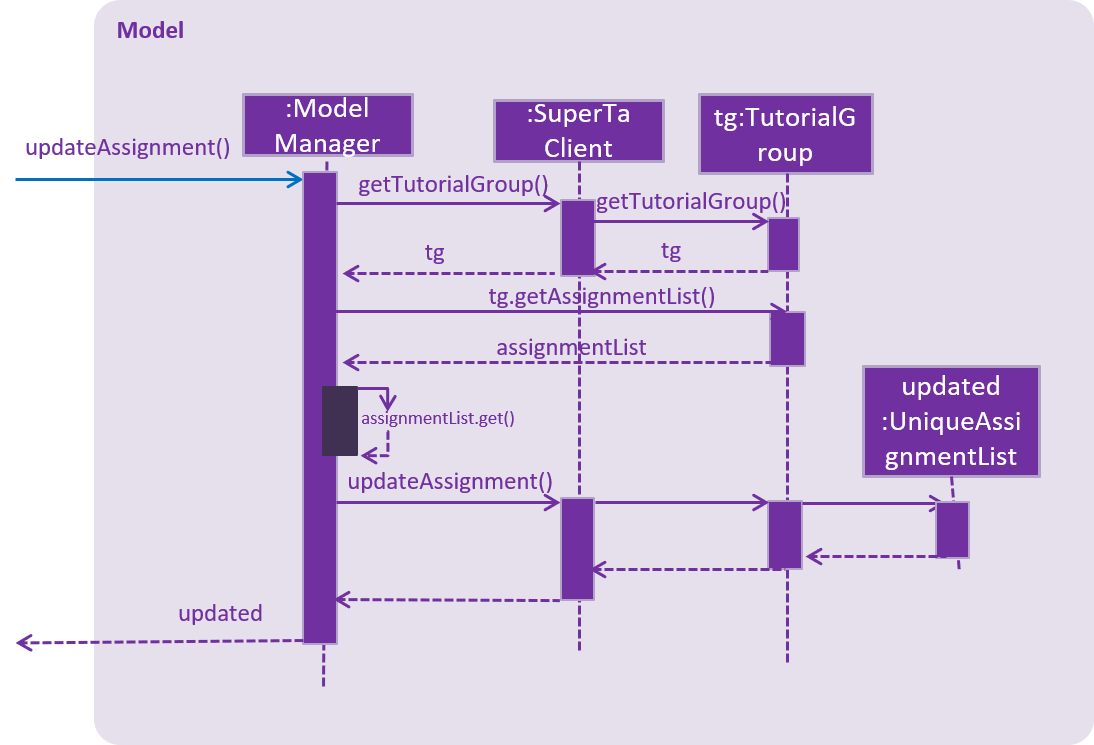
update-assignment
command-
When
updateAssignment()
is called inModelManager
, it will retrievetutorialGroup
andassignment
information fromSuperTaClient
andTutorialGroup
respectively. -
After locating the specific
assignment
to be changed,ModelManager
now callsupdateAssignment()
toUiqueAssignmentList
to replace the old assignment details. -
Updated information will be returned to the
logic
component (as ‘updated’) in the above diagram.
Design Considerations
Aspect: Implementation of UpdateAssignmentDescriptor
in UpdateAssignmentCommand
-
Alternative 1 (current choice): Use of
UpdateAssignmentDescriptor
inUpdateAssignmentCommand
to store the updatedassignment
details.-
Pros: Strengthen Single Responsibility Principle (SRP), as the
UpdateAssignmentCommand
will not need to check for differences between the old and updatedassignment
details. -
Cons: May increase coupling. If the additional
assignment
details are added in in the future such as section maximum marks,UpdateAssignmentDescriptor
have to change accordingly as well.
-
-
Alternative 2: Remove
UpdateAssignmentDescriptor
and parse updatedassignment
details as a newassignment
.-
Pros: Decreases coupling.
-
Cons: Harder to implement if user only indicate to update one field. (eg: update
assignmentTitle
only, whilemaxMarks
stay the same). In this case,UpdateAssignmentCommand
need to factor in the differences changed after the updatedassignment
details.
-
Aspect: Data structure of update-assignment
in ModelManager
-
Alternative 1: Locate
assignment
fromassignmentList
, then delete oldassignment
and add updatedassignment
.-
Pros: Easier to implement as it made use of other existing assignment commands such as
addAssignment()
anddeleteAssignment()
. No additional methods are needed forupdateAssignment()
. -
Cons: As each assignment contains a
GradeBook
which stores all student grades, deleting the oldassignment
and add a newassignment
may risk of losing the existingGradeBook
records. In addition, assignment index list may be affected after deletion as well.
-
-
Alternative 2 (current choice): Locate
assignment
fromassignmentList
and replace the oldassignment
with updatedassignment
details.-
Pros: No risk of losing
GradeBook
records as only theassignmentTitle
andmaxMarks
are being edited. -
Cons: An additional method,
updateAssignment()
will be implemented instead.
-
Finding a student
-
Finding a student while all students are listed
-
Test case:
find n/john
Expected: All students with namejohn
will be on the list. Since it is case-insensitive, variations such as ‘John’ or ‘joHn’ will appear on the filtered list as well. -
Test case:
find n/john mary
Expected: All students with namejohn
or ‘mary’ will be on the list. Similar to a, case-insensitive variations will appear on the filtered list. -
Test case:
find p/91234567 id/A0123456T
Expected: All students with phone number91234567
or with student IDA0123456T
will appear on the filtered list. -
Other than the indicated prefixes (
n/
,p/
, ‘e/’, ‘id/’), any other prefixes (ie.tg/
, ‘m/`) will show an invalid command format error message.
-
Updating an assignment detail
-
Update an assignment detail
-
Prerequisites: Have one tutorial group with an ID of
04a
.
View the tutorial group using theview-tutorial-group id/04a
command.
Have an assignment title namedlab1
with a maximum mark of40.0
.
Have an assignment title namedlab3
with a maximum mark of50.0
. -
Test case:
update-assignment tg/04a as/lab1 new_as/lab2
Expected: Assignmentlab1
name should now be changed tolab2
under theAssignments
UI panel. Maximum marks should be maintained at40.0
marks. -
Test case:
update-assignment tg/04a as/lab2 new_m/50.0
Expected: Assignmentlab2
maximum marks should be changed from40.0
marks to50.0
marks under theAssignments
UI panel. -
Test case:
update-assignment tg/04a as/lab2 new_m/lab3
Expected: An error message should be displayed, showing that assignment name already exists in the database. -
Test case:
update-assignment tg/04a as/lab4 new_m/lab5
Expected: An error message should be displayed, showing that assignment does not exist.
-